Reactを学習することになったのでチュートリアルをやってみようと思う。しかし、チュートリアルはTypescriptではないので、調べながら変更していこうと思う。
前提
- Node.js v16.15.0
- React v18.1.0
- Windows 10
チュートリアルの準備
ローカル開発環境
- 最新のNode.jsがインストールされていることを確かめる。
node -v
v16.15.0
- Create React App のインストールガイドに従って新しいプロジェクトを作成する。
npx create-react-app react-tutorial-typescript --template typescript
インストールされたもの
"dependencies": {
"@testing-library/jest-dom": "^5.16.4",
"@testing-library/react": "^13.2.0",
"@testing-library/user-event": "^13.5.0",
"@types/jest": "^27.5.0",
"@types/node": "^16.11.34",
"@types/react": "^18.0.9",
"@types/react-dom": "^18.0.3",
"react": "^18.1.0",
"react-dom": "^18.1.0",
"react-scripts": "5.0.1",
"typescript": "^4.6.4",
"web-vitals": "^2.1.4"
},
src/
フォルダ内のindex.css
を、以下のCSSコードで上書きする。
body {
font: 14px "Century Gothic", Futura, sans-serif;
margin: 20px;
}
ol, ul {
padding-left: 30px;
}
.board-row:after {
clear: both;
content: "";
display: table;
}
.status {
margin-bottom: 10px;
}
.square {
background: #fff;
border: 1px solid #999;
float: left;
font-size: 24px;
font-weight: bold;
line-height: 34px;
height: 34px;
margin-right: -1px;
margin-top: -1px;
padding: 0;
text-align: center;
width: 34px;
}
.square:focus {
outline: none;
}
.kbd-navigation .square:focus {
background: #ddd;
}
.game {
display: flex;
flex-direction: row;
}
.game-info {
margin-left: 20px;
}
src/
フォルダ内のindex.tsx
からreportWebVitals
に関する記述を削除する。
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
//import reportWebVitals from './reportWebVitals'; // ←削除する
const root = ReactDOM.createRoot(
document.getElementById('root') as HTMLElement
);
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
//reportWebVitals(); // ←削除する
src
フォルダにSquare.tsx
ファイルを作成する。
const Square = () => {
return (
<button className="square">
{/* TODO */}
</button>
);
};
export default Square;
src
フォルダにBoard.tsx
ファイルを作成する。
import Square from "./Square";
const Board = () => {
const renderSquare = (i: number) => {
return <Square />;
};
const status = 'Next player: X';
return (
<div>
<div className="status">{status}</div>
<div className="borad-row">
{renderSquare(0)}
{renderSquare(1)}
{renderSquare(2)}
</div>
<div className="borad-row">
{renderSquare(3)}
{renderSquare(4)}
{renderSquare(5)}
</div>
<div className="borad-row">
{renderSquare(6)}
{renderSquare(7)}
{renderSquare(8)}
</div>
</div>
);
};
export default Board;
src
フォルダのApp.tsx
ファイル書き換える。
import Board from './Board';
const App = () => {
return (
<div className='game'>
<div className='game-board'>
<Board />
</div>
<div className='game-info'>
<div>{/* status */}</div>
<ol>{/* TODO */}</ol>
</div>
</div>
);
};
export default App;
- プロジェクトフォルダ内で
npm start
を実行し、ブラウザでhttp://localhost:3000
を開くと空の三目並べの盤面が表示される。
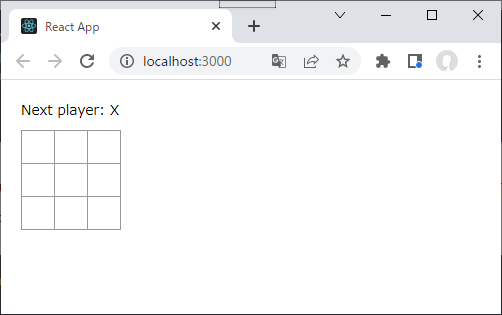
コメント